As I sat at my desk, staring at the lines of code, I felt awe and wonder. JavaScript, the language I’d worked with for years, held a secret power. This power, known as closures, changed how I approached web development, bringing efficiency, flexibility, and security.
At first, closures seemed daunting as a beginner. But I was determined to learn. I knew mastering closures could open up new possibilities. So, I started exploring these remarkable language features, eager to share what I learned with others.
In this guide, we’ll cover the basics of JavaScript closures. We’ll look at their real-world uses and the benefits they bring to web development. This article is for everyone, whether you’re experienced or just starting. It will help you use closures to improve your JavaScript skills.
Key Takeaways
- Understand the fundamental concepts of JavaScript closures and how they work.
- Discover the practical applications of closures in real-world web development scenarios.
- Explore the benefits of using closures, including data privacy, encapsulation, and performance optimization.
- Learn the basics of scope and lexical scope in JavaScript, which are crucial for understanding closures.
- Gain insights into advanced closure techniques, such as currying and function factories.
What Are JavaScript Closures and Why They Matter
In the world of understanding javascript closures: a beginner’s guide, closures are key. A closure is a function that can use variables from an outer function, even after that function is done.
The Core Concept of Closures
Closures are special because they let a function remember its surroundings, even when those surroundings are gone. This helps in making private variables and keeping data safe. It’s a big deal for data privacy and keeping things organized.
Real-World Applications of Closures
Closures are used in many ways, like making private parts of code and managing state in web apps. They’re great for private variables and keeping data safe. This is super important for web development today.
Benefits for Modern Web Development
Closures are super useful in web development today. They help make self-contained, reusable code and keep data safe. This leads to stronger, bigger, and easier-to-maintain web apps.
Learning about understanding javascript closures: a beginner’s guide opens up new doors for developers. It lets them write better, more secure code.
The Fundamentals of Scope in JavaScript
As a web developer, knowing about scope in JavaScript is key. Scope is about where and when you can use variables in your code. It’s vital for making your JavaScript closures work right.
JavaScript has three main scope types: global scope, function scope, and block scope. Global scope means variables are accessible everywhere. Function scope limits access to the function where they’re defined. Block scope, introduced in ES6, keeps variables in curly braces, like in if
or for
loops.
It’s also important to understand lexical scope in JavaScript. Lexical scope is about the scope a function has when it’s created, based on its location in the code. This is closely tied to JavaScript closures, which let functions use variables from outside, even after they’re done.
“Scope in JavaScript is fundamental to how the language works and is critical to understanding how to write maintainable and effective code.”
Learning about the different scope types and their role in JavaScript closures will help you become a skilled JavaScript developer. You’ll be able to write efficient and solid code.
Understanding JavaScript Closures: A Beginner’s Guide
Exploring JavaScript, closures become key. They are essential for advanced coding. We’ll cover the basics, create a closure, and see when to use them.
Basic Closure Syntax
A closure is a function that uses variables from another function. This happens when the inner function keeps using the outer function’s variables. Here’s what a basic closure looks like:
function outerFunction() {
const outerVar = 'I am outside!';
function innerFunction() {
console.log(outerVar); // We can access outerVar here
}
return innerFunction;
}
const myInnerFunction = outerFunction();
myInnerFunction(); // Logs 'I am outside!' to the console
Creating Your First Closure
To make your first closure, start with an outer function. This function should have variables you want to keep. Then, create an inner function that uses these variables. Finally, return the inner function from the outer one. This lets you call it later and use the variables.
Common Use Cases
Closures are useful in many ways in JavaScript. They help with private variables, persistent state, and more. Here are some examples:
- Emulating private variables and methods
- Creating persistent state in a web application
- Building versatile callback functions
- Implementing memoization for performance optimization
- Constructing function factories and partial application
Learning about JavaScript closures helps you write better code. It’s a crucial skill for any JavaScript developer.
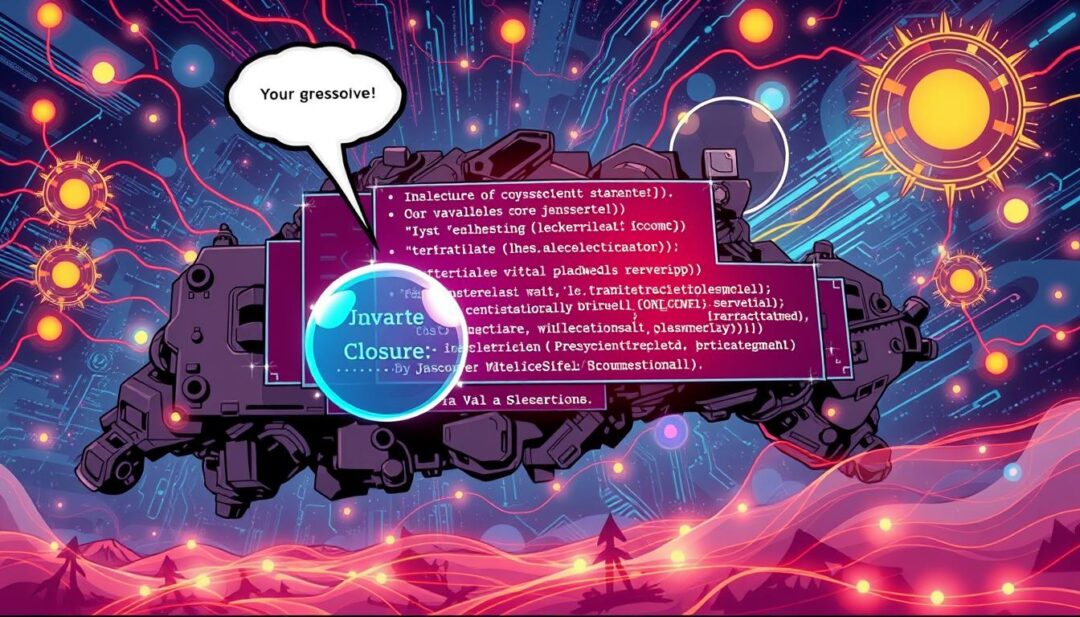
Working with Private Variables Through Closures
As a JavaScript developer, keeping data safe is key. Closures help us do this by creating private variables. This makes our apps more secure and reliable.
Data Privacy Techniques
Closures in JavaScript hide data from the outside. They do this by using a function inside another. This way, variables stay hidden and safe.
Encapsulation Methods
To keep data private, we use closures. We make a function that returns another. This inner function can see the outer function’s variables, even after it’s gone. This lets us keep variables safe and only let them be seen by the inner function.
Technique | Description | Example |
---|---|---|
Module Pattern | Encapsulates private data and methods within a single object, exposing only the desired public interface. |
const myModule = (function() { const privateVariable = 'secret'; return { publicMethod: function() { console.log(privateVariable); } }; })(); |
Immediately Invoked Function Expression (IIFE) | Defines a function and immediately invokes it, creating a private scope for variables and methods. |
const counter = (function() { let count = 0; return { increment: function() { count++; }, getCount: function() { return count; } }; })(); |
Best Practices for Variable Protection
Working with private variables through closures needs careful handling. Here are some tips:
- Don’t let private variables be seen directly. Use public methods to control access.
- Make sure closures don’t let others mess with your variables by accident.
- Use tools to check your code for any security issues with private variables, data privacy, and closures in loops.
- Write clear comments to explain what your private variables and closures do.
Learning to use closures for private variables makes your JavaScript apps safer. It keeps sensitive info safe and your code solid.
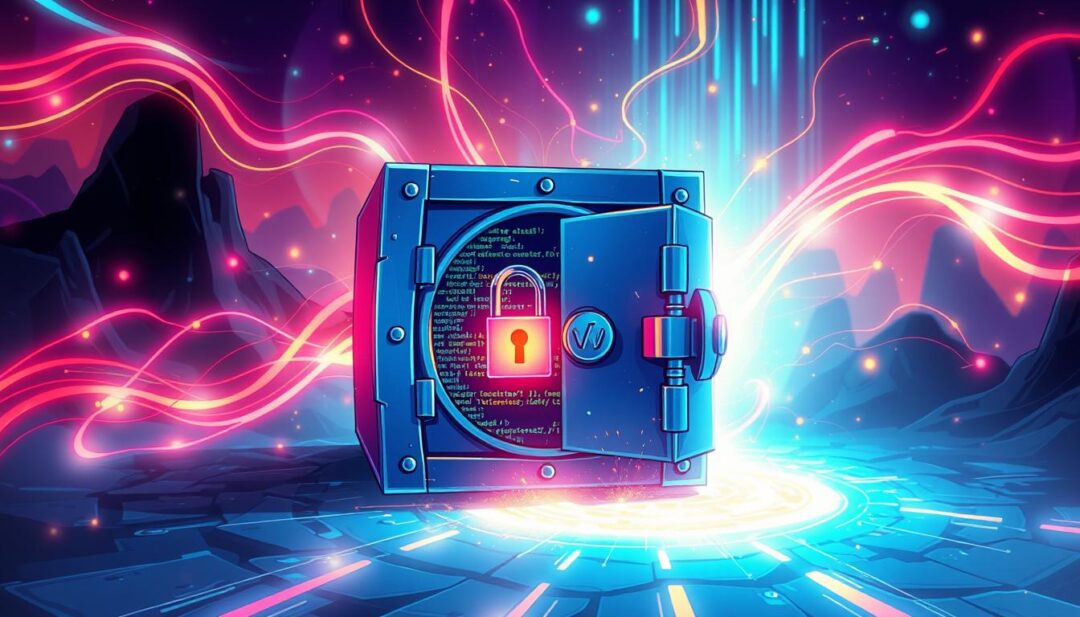
Advanced Closure Techniques: Currying and Function Factories
As I dive into JavaScript closures, I find two key techniques: currying and function factories. These methods make our code more efficient and flexible. They help solve common problems, making our code easier to read and maintain.
Implementation of Currying
Currying changes a function that takes many arguments into a series of functions. Each function takes only one argument. This makes our code more modular and easy to use.
I’ll show how currying lets us make specific functions from general ones. This improves our code’s readability and flexibility.
Creating Efficient Function Factories
Function factories are another powerful tool. They let us create functions that can be reused for specific tasks. By using closures, we can make functions on demand, tailored to our needs.
I’ll explain how function factories work. They help avoid memory leaks and improve performance, especially with dynamic data.
Performance Optimization Tips
While exploring these techniques, I’ll share tips for better performance. I’ll talk about avoiding memory leaks and how to fix them. Understanding closures helps keep our code efficient and scalable, even with complex applications.
FAQ
What are JavaScript closures and why do they matter?
A JavaScript closure is a function that can use variables from an outer function, even after that function ends. They are key in web development. They help keep data safe, make code reusable, and support advanced coding techniques.
How do the concepts of scope and lexical scope relate to JavaScript closures?
Scope in JavaScript is about where variables and functions can be used. Lexical scope is about how this is decided based on where they are in the code. Knowing about scope and lexical scope helps you understand and use closures well.
What are some real-world applications of JavaScript closures?
Closures are used in many ways in JavaScript. They help keep data safe, make code reusable, and support advanced coding. They are great for creating modular and efficient code, especially in complex apps.
How can I create a simple closure in JavaScript?
To make a closure, create an inner function that can use variables from an outer function. This inner function can then use and change those variables even after the outer function ends. This is a basic but powerful concept in JavaScript.
How can I use closures to create private variables and achieve data privacy?
Closures are good for making private variables and keeping data safe in JavaScript. By using an outer function to define variables and an inner function to access them, you can keep data private. This is a common way to protect data in JavaScript apps.
What are some advanced closure techniques, such as currying and function factories?
Closures also support advanced techniques like currying and function factories. Currying lets you make specialized functions by adding arguments one at a time. Function factories create new functions with specific abilities. These methods use closures to make code more flexible and efficient.
How can I avoid potential memory leaks when working with closures?
Closures can sometimes cause memory leaks if they hold onto variables that are no longer needed. To avoid this, manage the life cycle of variables in closures carefully, especially in loops or event handlers. Properly releasing references helps keep your app’s memory usage low.
Leave a Reply